The | (bitwise OR) in C or C++ takes two numbers as operands and does OR on every bit of two numbers. The result of OR is 1 if any of the two bits is 1.
OR gate:
Example 2:
When the binary value for a ( 0101 ) and the binary value for b ( 1100 ) are OR 'ed together we get the binary value of
1101 :
int a = 0 1 0 1
int b = 1 1 0 0
---------
int c = 1 1 0 1
Decimal to Binary conversion :
Step 1: Divide the given decimal number by 2 and note down the remainder.
Step 2: Now, divide the obtained quotient by 2, and note the remainder again.
Step 3: Repeat the above steps until you get 0 as the quotient.
Step 4: Now, write the remainders in such a way that the last remainder is written first, followed by the rest in the reverse order.
Example :
Lets take the decimal number 13 :
13÷2 = 6 ---> Reminder ---> 1
6÷2 = 3 ---> Reminder ---> 0
3÷2 = 1 ---> Reminder ---> 1
1÷2 = 0 ---> Reminder ---> 1
13 in binary = 1101
Binary to Decimal conversion :
Converting number : 11000100
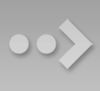
=196
Decimal to Binary Table :